php table source code
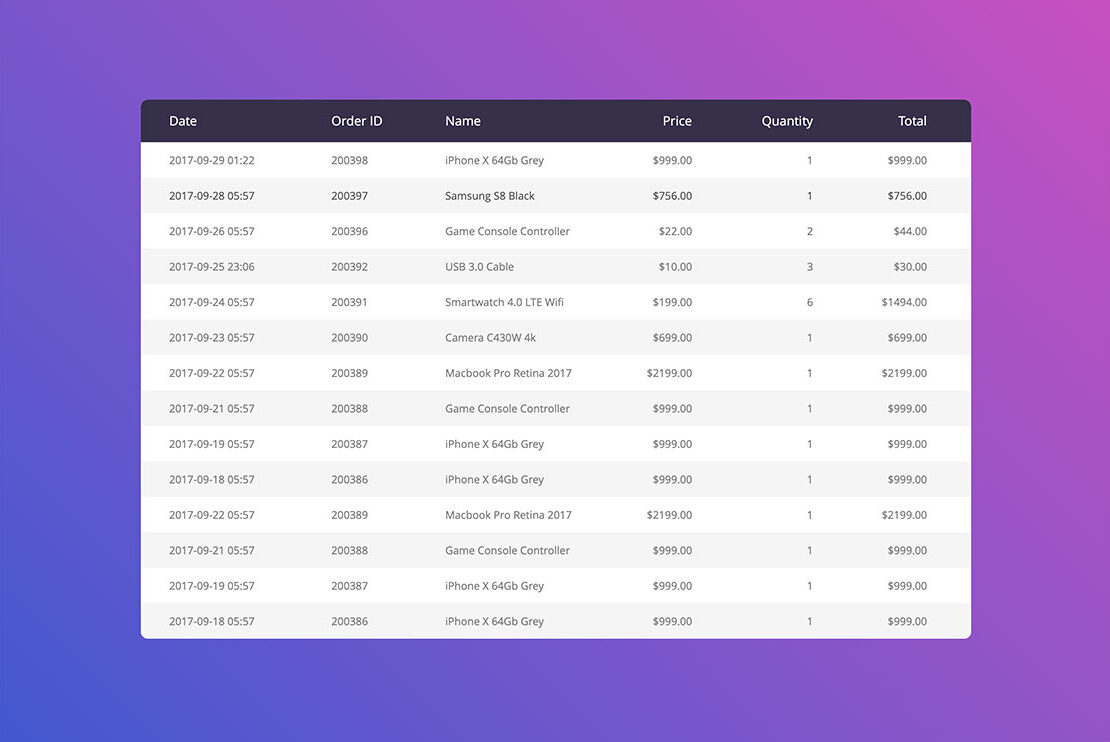
Introduction:
Tables are fundamental components in web development, enabling developers to organize and present data in a structured format. In PHP, creating and managing tables involves generating dynamic content that can be fetched from databases or other data sources. This tutorial will guide you through the process of creating a PHP table, demonstrating how to display data using Tables are particularly useful for displaying records, such as user information, product details, or any other type of structured data. By leveraging PHP, you can create tables that dynamically populate based on data retrieved from a database. This capability is essential for creating interactive and data-driven web applications. Using PHP in conjunction with SQL queries allows you to fetch and display data efficiently, providing a seamless user experience.HTML and PHP, and incorporating CSS for styling. php table source code
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PHP Table Example</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 0;
}
.container {
width: 80%;
margin: 20px auto;
}
table {
width: 100%;
border-collapse: collapse;
margin: 20px 0;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
table th, table td {
padding: 12px;
text-align: left;
border-bottom: 1px solid #ddd;
}
table th {
background-color: #f4f4f4;
}
table tr:nth-child(even) {
background-color: #f9f9f9;
}
table tr:hover {
background-color: #f1f1f1;
}
.container h1 {
text-align: center;
color: #333;
}
</style>
</head>
<body>
<div class="container">
<h1>PHP Table Example</h1>
<?php
// Database connection (replace with your own credentials)
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "test_db";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SQL query to fetch data from the table
$sql = "SELECT id, name, email, registration_date FROM users";
$result = $conn->query($sql);
// Check if there are results
if ($result->num_rows > 0) {
// Output data of each row
echo "<table>";
echo "<tr><th>ID</th><th>Name</th><th>Email</th><th>Registration Date</th></tr>";
while($row = $result->fetch_assoc()) {
echo "<tr>";
echo "<td>" . $row["id"] . "</td>";
echo "<td>" . $row["name"] . "</td>";
echo "<td>" . $row["email"] . "</td>";
echo "<td>" . $row["registration_date"] . "</td>";
echo "</tr>";
}
echo "</table>";
} else {
echo "<p>No records found.</p>";
}
// Close the connection
$conn->close();
?>
</div>
</body>
</html>
Conclusion
Creating tables with PHP is a powerful way to present structured data on your website. This tutorial has walked you through the essential steps of designing a PHP table using HTML for structure, CSS for styling, and PHP for dynamic data handling. By leveraging these technologies, you can build tables that not only display data effectively but also adapt to changes in your In summary, you have learned how to set up a table layout with HTML, enhance its appearance with CSS, and populate it with data from a database using PHP. This approach ensures that your tables are functional, aesthetically pleasing, and capable of handling dynamic content.database.
Facebook
Twitter
LinkedIn
WhatsApp
Email
X
Print