php login form source code
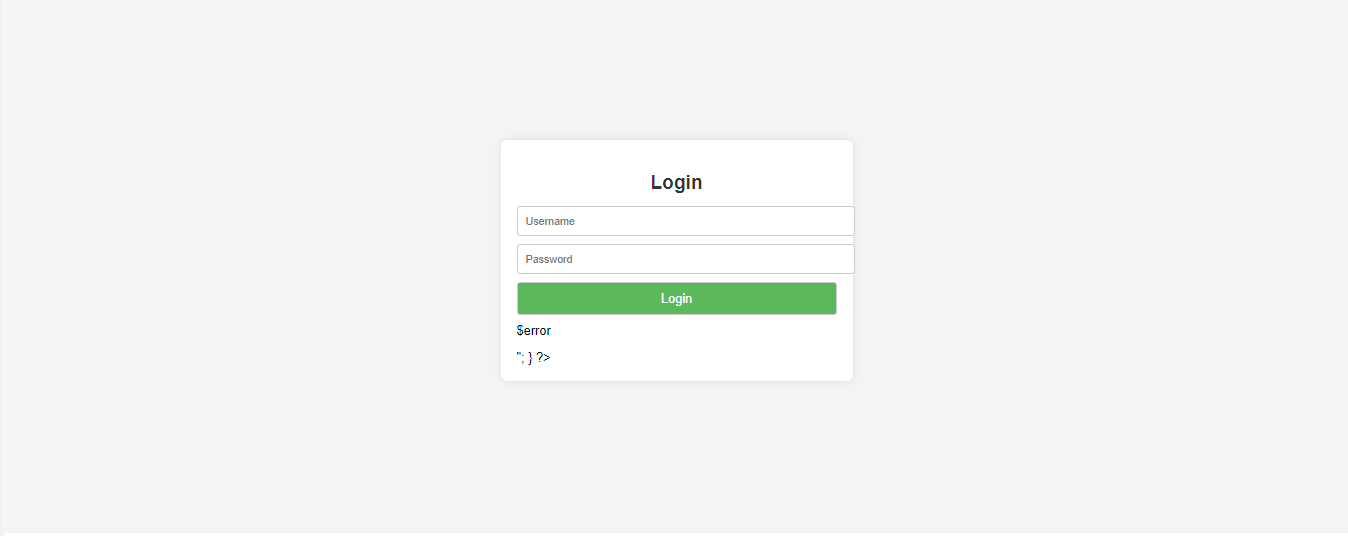
Introduction:
A PHP login form is a crucial component of any secure website, providing users with access to protected content and personalized features. Whether you’re building a simple blog, an e-commerce site, or a complex web application, implementing a login system is essential for managing user authentication and protecting sensitive data. In this tutorial, we’ll guide you through the process of Creating a login form involves several important steps, including setting up the HTML form fields, applying CSS to create a user-friendly interface, and writing PHP scripts to validate user credentials. HTML provides the basic structure, including fields for the username and password. CSS enhances the visual appeal, ensuring that the form is easy to use and visually consistent with the rest of your website. PHP, the backbone of the login system, handles the authentication process by verifying the user’s credentials against a database.creating a PHP login form using HTML for structure, CSS for styling, and PHP for handling authentication logic.php login form source code
HTML Code
<?php
// Database connection
$host = 'localhost';
$db = 'your_database_name';
$user = 'your_database_user';
$pass = 'your_database_password';
$conn = new mysqli($host, $user, $pass, $db);
if ($conn->connect_error) {
die('Connection failed: ' . $conn->connect_error);
}
// Handling form submission
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$email = $conn->real_escape_string($_POST['email']);
$password = $_POST['password'];
$sql = "SELECT * FROM users WHERE email = '$email'";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
$user = $result->fetch_assoc();
if (password_verify($password, $user['password'])) {
echo 'Login successful!';
// Here you could start a session and store user info
} else {
echo 'Invalid password.';
}
} else {
echo 'No user found with that email address.';
}
}
$conn->close();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login Form</title>
</head>
<body>
<form action="" method="POST">
<label for="email">Email:</label><br>
<input type="email" id="email" name="email" required><br><br>
<label for="password">Password:</label><br>
<input type="password" id="password" name="password" required><br><br>
<input type="submit" value="Login">
</form>
</body>
</html>
Conclusion
Creating a PHP login form is a vital step in building a secure and user-friendly website. Throughout this tutorial, we’ve covered the essential steps to design and implement a login system using HTML, CSS, and PHP. From structuring the form with HTML, enhancing its appearance with CSS, to writing the PHP code for validating user credentials, you now have a complete This login form not only protects sensitive content on your website but also provides a seamless user experience. By following the steps outlined, you’ve ensured that only authorized users can access protected areas of your site, enhancing both security and user satisfaction. Whether you’re a beginner or an experienced developer, this tutorial equips you with the necessary skills to implement a robust authentication system.understanding of how to create a functional and secure login form.php login form source code
Facebook
Twitter
LinkedIn
WhatsApp
Email
X
Print